- You are rather well to do with AS3
- You have already looked through my previous tutorials
- You know how to setup and already installed Flash Develop
- You have already link to the Flex SDK (FREE download btw) and compiled the 'Hello World' example I previously wrote about.
-
-
Ok.. here I am also assuming that
- You want to program a game
- You want to program a 2D game
- You want to program a 2D game with sprites
- You want to program a 2D game with sprites in AS3
-
If you are looking for 3D Flash, look elsewhere or maybe look here in like a few years later. I know we already have Papervision and other stuffs but I am still trying to grab 2D.
First I will need you to download a game library... it will be Flixel.. yeah... Wha? Why not Flashpunk?
Well err... currently I have already started with Flixel.
Ok the reason being that flixel have less letters to type. There!
Start an 'AS3 Project' under Flash Develop. Flash Develop will generate those default files. I call my project name Ship. What we are going to do is to :
- Add a AS3 Project
- Setup the Flixel Library
- Initialises the FlxGame and FlxState
- View the prebuilt in features
After creating a new project, you will get a Main.as default file and if you press 'F5' to test the movie, it will be a blank screen.
Now it will look like this in the Main.as.
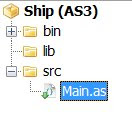
Clear all those texts and import stuffs because we are going to use flixel and when we use flixel, flixel handles the import for us in the library itself
package
{
import org.flixel.*;
[SWF(width = "500", height = "350", backgroundColor = "#000000")]
public class Main extends FlxGame
{
public function Main():void
{
super(500, 350, LoadMeShip, 1);
}
}
}
Just copy whatever is in above and replace whatever which was in the Main.as with it. There... hit 'F5'. You got a game running! Yeah!
...
..
.
Sadly no... it's not that easy.
You did download the flixel library already right? Just unzip it and
It should look like this in the Project view.
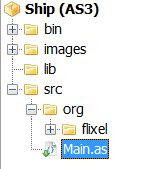
Ok now that you have copied the items into the correct place, hit 'F5' Wha? Again? You should get an error stating some undefined properties LoadMeShip. It's ok.

Let's go line by line on the code we just put in.
import org.flixel.*;
- This simply means we are importing the flixel library located at org/flixel folder relative to the main.as and * simply means everything. So it translate to 'gimme everything from org/flixel'
[SWF(width = "500", height = "350", backgroundColor = "#000000")]
- Since we don't have a nice interface to tell how big we want the flash movie to be like in Flash Pro, we have to do it here like this. The parameters should explain themselves. Or should they?
public class Main extends FlxGame
- Why? extends? What? The class name 'Main' directly relates the the filename Main.as. When compile, the system will look for the filename as the class name so basically this is the entry point.
- extends? If you have some kind of programming background this is equivalent to inherit. I hope I am right but basically this means the Main class inherits the FlxGame class (which by the way is located in org/flixel/FlxGame.as) and is capable of extending it's functionality. Gosh correct me if I am wrong...
- If you look into FlxGame.as you will see it imports flash.display.Bitmap, Sprites and all so basically this FlxGame imports those stuffs and when you import extends FlxGame.as you kinda use those too.
- Ok why do we need to extend from FlxGame? Well this is the kinda core class to extends from when using Flixel. To actually learn more about this, you can view in the documentation that comes with the downloaded library under docs/index.html. You will need to refer to these docs VERY frequently as I have to really understand the library you are using. It helps. It really does... and if you are wondering... wow.. these documents are cool.. how do they do that and keep up with all the updates. The answer is 'You can do it too :D' In Flash Develop, look under Tools->Flash Tools->Documentation Generator... wait I am going off topic... later then.
public function Main():void
- Hmm.. how do I explain this. This is actually the constructor function of the class. A function with the same name as the class will be it's constructor, the one that executes upon initialisation of the class
- This is what will run first. As a further reading for you guys, read up on destructor.
super(500, 350, LoadMeShip, 1);
- No relation to Superman, try to guess what it does?
- Although not immediately clear, the 'super' actually calls the class's superclass's constructor!
- Yes... Main being an extention of FlxGame, so Main's superclass is actually FlxGame class and FlxGame constructor is actually the FlxGame function in the FlxGame class which is in FlxGame.as file in org/flixel folder :p. Get it? I really hop you do because, there will be lots of super calling in the future and I really hope you know what it actually means.
- Note the parameter passed in and relate it to the FlxGame constructor function : FlxGame(GameSizeX:uint,GameSizeY:uint,InitialState:Class,Zoom:uint=2)
- 500 = GameSizeX:uint (Width of hte game screen)
- 350 = GameSizeY:uint (Height of the game screen)
- LoadMeShip = InitialState:Class (It will look for the LoadMeShip class, in this case, it's not found cause we have not add it yet!)
- 1 = Zoom:uint=2 (Zoom level with a default of 2)
So now that you realised the error came from the missing LoadMeShip class, all you need to do is to add that class in. Right click on the 'src' folder and Add->New Class
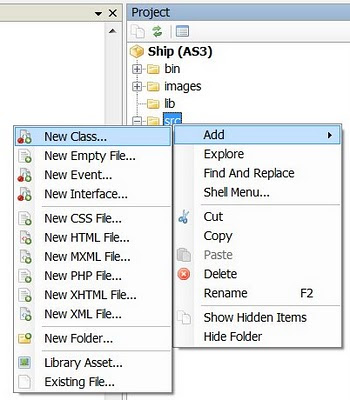
Call it 'LoadMeShip'. Again we don't want the default items in the class so just clear them out and replace with ...
package
{
import org.flixel.FlxState;
public class LoadMeShip extends FlxState
{
public function LoadMeShip():void
{
trace('I am running');
}
}
}
FlxState? What's that? Lets just put it as errr.... game states. You know like menu screen, level 1, level 2... so basically it's a game state we are dealing with here.
You can read more about it in the docs. Now hit() 'F5' to run the test movie. If you get the
You should already know how to solve this (for Flex SDK 4) in my previous post. Just add
in the Project->Properties->Compiler Options->Additional Compiler Options
Everything should run fine now. If you are fast enough you can catch the volume control sliding up out of view. This is a default for the volume control. You have lots of other prebuilt stuffs too. Try pressing '`'. You get a console telling your framerate and other infos. If you lose focus on the flash window, your game pauses and a pause screen shows :D
So basically these are the stuffs which are handled by the Flixel game library.
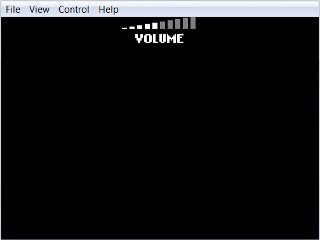
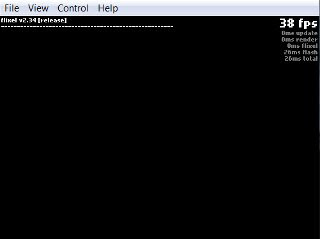
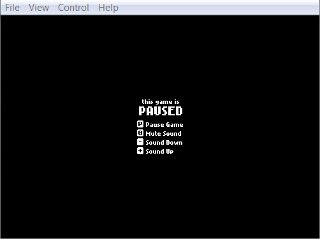
You should also be able to see the 'I am running' in the output panel in Flash Develop! If not you might be using a non debug flash player (solution also explain in my previous blog entry).
Ok that's about as much I am able to write here for today. Sleeping already? Yes it's kinda boring at first but all these needs to be done for the progress to the next tutorial.
7 comments:
Yo, post this shizat up at the Github (http://wiki.github.com/AdamAtomic/flixel/)
and in the forums: mo people will read this shizat
Ok I will try :D Thanks
That was amazing, thank you!
Perfect for me the nubie
Thanks for the tutorial, it was useful. Too bad you spent so much time trying to be cute, only to cut off when things were actually getting interesting. At least I learned how to set up Flixel, if not how to use it. If you write more tutorials, I'll read them. :)
Cheers.
Haha thanks for this, and you're pretty funny even now in 2013.
What do you use to open up the game screen? I tried Chrome but I just got a blank, white screen and no volume button anywhere. Your suggested key presses didnt show up either.
Post a Comment