Create a new Flash File. Draw a simple
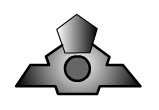
Not much of a
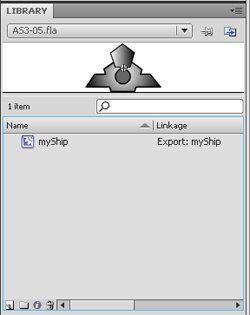
Now delete the original shape on stage. Yes… delete it! Select it and delete. Don’t worry… it has been immortalized in the form of a symbol in the library… well until you delete the symbol.. hmmm. Ok now the stage is empty. We will use AS3 to fill it with the
Right click and select ‘Action’ on Frame1 to bring up the ActionScript Editor. (Actually this is not the best way to do this… I will explain in future
var clownShip:myShip = new myShip; //yes… the name clownship is catching on!!!
addChild(clownShip);
‘//’ Simply puts the characters after ‘//’ as comment until the end of line. It’s good for
You can test the movie now by pressing ‘CTRL+ENTER’. Your clownship appears to be on top left corner of the stage. Position it near bottom middle…
clownShip.x = stage.stageWidth/2;
clownShip.y = stage.stageHeight - 40;
Now this will cause a little overhead to the processing power. Why? Because we could have set the x (horizontal) and y (vertical) position of the clownShip before we add it into the stage (addChild). Currently we add it to stage (default at 0,0) then move it to half of the stage’s width and 40 pixel from the bottom of the stage.. meaning the flash player needs to first position it at 0,0 and then reposition it at stageWidth/2, stage.stageHeight-40…. 2 steps of rendering the
We could go around this by re-arranging the codes as below
var clownShip:myShip = new myShip;
clownShip.x = stage.stageWidth/2;
clownShip.y = stage.stageHeight - 40;
addChild(clownShip);
This way, the flash player only needs to render the clownShip once at stageWidth/2, stage.stageHeight-40.
Big deal, we got tons of processing power… that’s what we all thought. But it is these small things that eat up the processing power itty bitty by itty bitty and if you loop the above statement by 1000 times, those will be an additional 1000 steps the flash player needs to take. Now,
stage.addEventListener(KeyboardEvent.KEY_DOWN, listenKey);The listener will call the function listenKey each time a key is pressed. Now lets put in the listenKey function…
function listenKey(event:KeyboardEvent)
{
if (event.keyCode == 32) {
trace('I have pressed the spacebar');
}
}
This function receives keyboard event and if you look carefully the keyboard keys are referred to by numbers. It’s ok, you just need to memorize all keys and their corresponding key code (keyCode). For example, the ‘spacebar’ holds the keyCode of 32, the ‘up arrow’ holds 38, down 40, left 37 and right 39. Now if you got a key which you would like to find out its key code, you can add this line to the code…
trace(event.keyCode);
…in the listenKey function. So basically the function would look like this…
function listenKey(event:KeyboardEvent)Try to test run the movie, remember to check the ‘Disable Keyboard Shortcut’ from the test movie window and press any key to see their corresponding key codes being displayed in the output (debug) window in the flash app. Hmm…. There is another thing called charCode instead of keyCode. Try reading about it in the help section.
{
trace(event.keyCode);
if
(event.keyCode == 32) {
trace('I have pressed the spacebar');
}
}
By now you should be able to know whichever key codes for any keys you wish to know to really want to know, you know. Now lets move on to moving the clown left and right via the keyboard. First you detect the left and right arrow keys…
function listenKey(event:KeyboardEvent)…then you add or minus from the current clown’s horizontal position… here…
{
if (event.keyCode == 32) {
}
if (event.keyCode == 37) { //Left arrow
}
if (event.keyCode ==
39) { //Right arrow
}
}
function listenKey(event:KeyboardEvent)
{
// trace(event.keyCode);
if (event.keyCode == 32) {
// trace('I have pressed the spacebar');
}
if (event.keyCode == 37) { //Left arrow
clownShip.x = clownShip.x
- 3
}
if (event.keyCode == 39) { //Right arrow
clownShip.x =
clownShip.x + 3
}
}
What happened here is I minus the x position of the clownShip by 3 pixels whenever I press the left key and add 3 pixels to it whenever I press the right key. What are pixels you say? They are
Now try to test run the movie. Move the clown around. Does it work? Well if it does, it is not looking good. A bit on the sluggish side I would say, especially on the start. Well, what went wrong?
1 comment:
You are totally right. This post actually made my day. You can not imagine just how much time I
had spent for this info! Thanks!
Splitwise
appvn
Post a Comment